Scope of the Variables
When a variable is declared in a
function then it remains local to that function. This means that, that
variable cannot be accessed from outside the function or by other
functions. It is helpful when two variables of same name have been
declared in different functions. This prevents you from accidentally
overwriting the contents of a variable.
In the following example you will see how a variable defined in a function cannot be accessed from outside the function.
Example |
<html> |
<body> |
<?php |
function abc() |
{ |
$a = "Testing this variable"; |
} |
echo "Lets test it: $a <br/>"; |
?> |
</body> |
</html> |
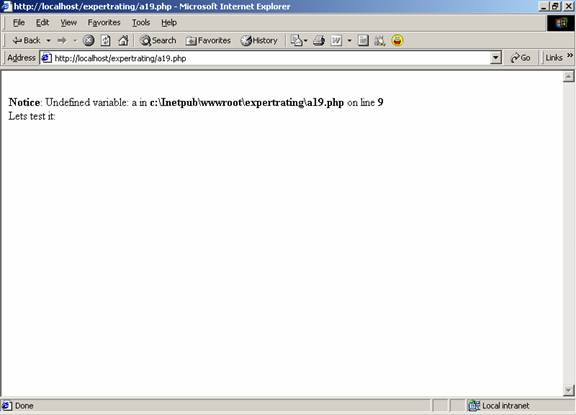
The global statement comes in handy
when you want to access variables that are declared outside the
function. In this if a variable is declared and assigned a value outside
a function, and then we declare a global variable of the same name in
the function, then that function can access the variable that has been
declared outside the function.
In the following example we will show
how a global variable declared inside a function can access the variable
declared outside the function.
Example: |
<html> |
<body> |
<?php |
$a = "Hello how are you"; |
function abc() |
{ |
global $a; |
echo $a; |
} |
abc(); |
?> |
</body> |
</html> |
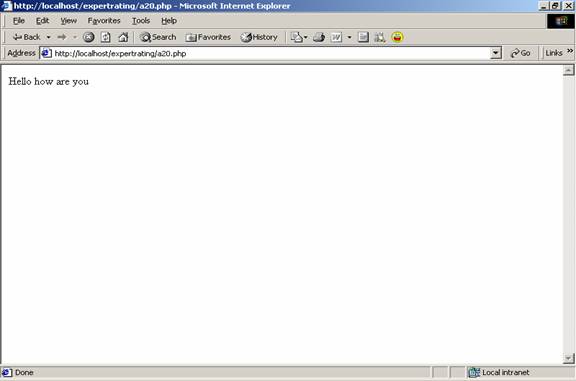
The Static Keyword
The static keyword is used when
declaring variables in functions. The purpose of the static variable is
to inform PHP that this variable should not be destroyed when the given
function executes. Instead of destroying that variable, the value of
that variable is saved for the next time needed.
The static variable is used to retain certain variables within the function for the life of the execution of the script.
Syntax |
Static $variable [= initial value]; |
In the following example we will be
using the static variable in the function to see that how many times a
given function has been called.
Example |
<html> |
<body> |
<?php |
function func() |
{ |
static $a = 0; |
$a++; |
echo "This function has executed $a time(s).<br/>"; |
} |
for($i=0; $i<8; $i++) |
{ |
func(); |
} |
?> |
</body> |
</html> |
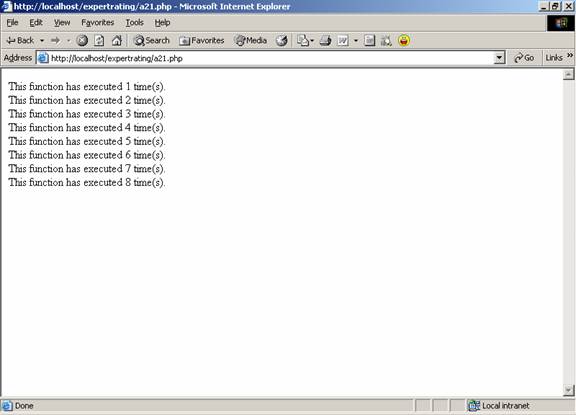
No comments:
Post a Comment